Slicing in Python
String Slicing in python is used to obtain the substring from the given string, also sub-list from the given list. You can also modify data using slicing. Slicing is used to remove or modify the data. Indexing plays an important role to perform slicing. Slicing in python can be done in two indexing methods such as Forward indexing or Backward indexing. In python Forward indexing start from 0. On other hand Backward indexing start from -1 on the right side.
Square brackets [] are used to perform a slicing operation in the list. In square brackets [] we have to give three parameters start, end, and step. Start, end, and step are declared according to user requirements. If the programmer won’t define the step then the step is 1 by default. It includes the start parameter, but it won’t include the end parameter. It takes end-1 instead of the end. So if you want to include n index values then you need to specify the n+1 index. The colon(:) is used to distinguish the start, end, and step parameters.
List Slicing in Python
A list slicing is used to perform useful operations such as reverse the list, obtain a sub-list.
Syntax:-
listName[ Start : end : step]
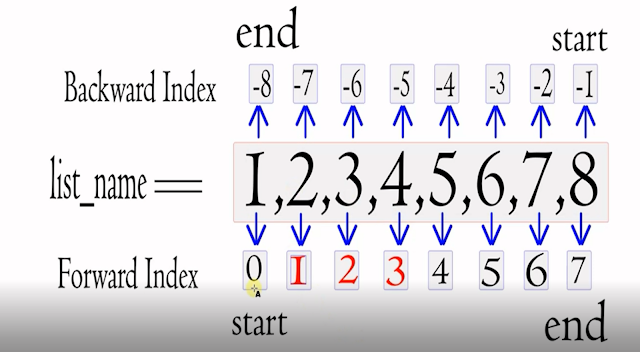
Examples:-
>> list = [1,2,3,4,5,6,7]
>>print(list)
Output:-
[1,2,3,4,5,6,7]
# If we want to print the first five elements
>> list = [1,2,3,4,5,6,7]
>>print(list[ : 6]) # No need to specify start, it automatically starts from index 0
Output:-
[1,2,3,4,5]
# If we want to print 2,3 and 4
>> list = [1,2,3,4,5,6,7]
# Now index of 2 is 1 i.e it starts from 1
# index of 4 is 3 but we know to include 3 we have to take 4. Let’s try.
>>print(list[1: 4])
Output:-
[2,3,4]
# If we want to print 5 and so on till the end then
>> list = [1,2,3,4,5,6,7]
# Index of 5 is 4
# Here no need to specify the value of the end parameter it automatically detects it
>>print(list[4 : ]) # No need to specify end, it automatically detects it
Output:-
[5,6,7]
# Print only odd numbers from the list
>> list = [1,2,3,4,5,6,7]
>>print(list[ : : 2]) # No need to specify start or end, it automatically detects it
Output:-
[1,3,5,7]
# Print only even numbers from the list
>> list = [1,2,3,4,5,6,7]
>>print(list[ 1: : 2]) # No need to specify start or end, it automatically detects it
Output:-
[2,4,6]
# Print the list in reverse order
>> list = [1,2,3,4,5,6,7]
>>print(list[ -1: : -1]) # No need to specify end, it automatically detects it
# Here we are moving from right to left i.e -1 + -1 = -2 and so on…
Output:-
[7,6,5,4,3,2,1]
String Slicing Python
String slicing is used to perform useful operations such as reverse the string, obtain a sub-string.
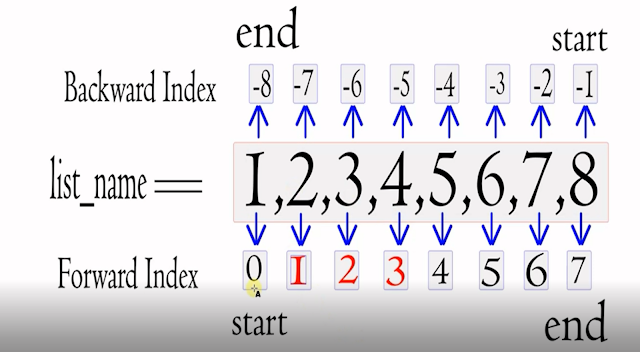
Syntax:-
stringName[ Start : end: step]
Examples:-
>> string = ‘my name is ram’
>> print(string)
Output:-
my name is ram
# Print only ram
>> string = ‘my name is ram’
>> print(string[-3 : ])
Output:-
ram
#Reverse the string
>> string = ‘my name is ram’
>> print(string[-1 : : -1])
Output:-
mar si eman ym
Hash(#) is used to comments the statements.
Post a Comment
Post a Comment